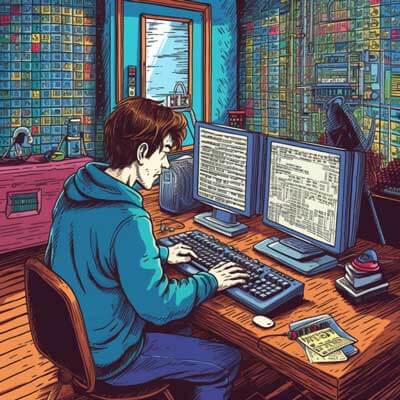
Table of Contents
Introduction to Python Generators
Python generators are a powerful feature that allows you to iterate over a collection of items or generate a sequence of values on the fly. Unlike traditional functions that return a value and then terminate, generators can pause and resume their execution, allowing for efficient memory usage and lazy evaluation.
Generators are defined using the yield
keyword, which is a special keyword in Python that allows a function to yield values one at a time instead of returning them all at once. This makes generators ideal for working with large or infinite sequences, as they only generate values as needed.
Related Article: How to Check If Something Is Not In A Python List
Understanding the Yield Keyword in Python
The yield
keyword is at the heart of Python generators and is used to define generator functions. When a generator function is called, it returns a generator object, which can then be used to iterate over the values generated by the function.
Each time the yield
keyword is encountered in a generator function, the function's execution is paused, and the current value is yielded. The next time the generator's __next__()
method is called, the function resumes execution from where it left off and continues until the next yield
statement is encountered.
This ability to pause and resume execution allows generators to produce values on-the-fly, making them memory-efficient and suitable for working with large datasets or infinite sequences.
Implementing Generators in Python
To define a generator function in Python, you simply use the yield
keyword instead of the return
keyword. Here's an example of a basic generator function that generates a sequence of even numbers:
def even_numbers(): num = 0 while True: yield num num += 2 # Using the generator even_gen = even_numbers() print(next(even_gen)) # Output: 0 print(next(even_gen)) # Output: 2 print(next(even_gen)) # Output: 4
In this example, the generator function even_numbers()
generates even numbers starting from 0. Each time the yield
statement is encountered, the current value of num
is yielded and the function's execution is paused. The generator object even_gen
can then be used to iterate over the generated values by calling the next()
function.
Code Snippet: Basic Generator Example
def count_down(n): while n > 0: yield n n -= 1 # Using the generator count_down_gen = count_down(5) for num in count_down_gen: print(num) # Output: 5, 4, 3, 2, 1
This code snippet demonstrates a simple generator function count_down()
that counts down from a given number. Each time the yield
statement is encountered, the current value of n
is yielded, and the function's execution is paused. The generator object count_down_gen
is then used in a for
loop to iterate over the generated values.
Related Article: How to Reverse a String in Python
Code Snippet: Generator with Conditional Statements
def even_numbers(): num = 0 while True: if num % 2 == 0: yield num num += 1 # Using the generator even_gen = even_numbers() print(next(even_gen)) # Output: 0 print(next(even_gen)) # Output: 2 print(next(even_gen)) # Output: 4
This code snippet demonstrates a generator function even_numbers()
that generates even numbers using a conditional statement. Only numbers that are divisible by 2 are yielded, ensuring that only even numbers are generated.
Code Snippet: Generator with Looping
def fibonacci(): a, b = 0, 1 while True: yield a a, b = b, a + b # Using the generator fib_gen = fibonacci() for _ in range(10): print(next(fib_gen)) # Output: 0, 1, 1, 2, 3, 5, 8, 13, 21, 34
This code snippet demonstrates a generator function fibonacci()
that generates Fibonacci numbers. The generator uses a loop to calculate the next Fibonacci number and yields it. The generator object fib_gen
is then used in a for
loop to iterate over the generated values.
Code Snippet: Generator with Error Handling
def safe_division(a, b): try: yield a / b except ZeroDivisionError: yield "Division by zero error occurred" # Using the generator div_gen = safe_division(10, 0) print(next(div_gen)) # Output: "Division by zero error occurred" div_gen = safe_division(10, 2) print(next(div_gen)) # Output: 5.0
This code snippet demonstrates a generator function safe_division()
that performs division and handles the ZeroDivisionError. If a ZeroDivisionError occurs, the generator yields the error message. Otherwise, it yields the result of the division.
Code Snippet: Generator with State
def counter(start): while True: yield start start += 1 # Using the generator counter_gen = counter(0) print(next(counter_gen)) # Output: 0 print(next(counter_gen)) # Output: 1 print(next(counter_gen)) # Output: 2
This code snippet demonstrates a generator function counter()
that generates an infinite sequence of numbers starting from a given value. The generator maintains its internal state by using a variable start
that is incremented on each iteration.
Related Article: How to Use Python dotenv
Advanced Techniques for Implementing Generators
Code Snippet: Generator with Multiple Yields
def even_odd_numbers(): num = 0 while True: if num % 2 == 0: yield num, "even" else: yield num, "odd" num += 1 # Using the generator even_odd_gen = even_odd_numbers() print(next(even_odd_gen)) # Output: (0, 'even') print(next(even_odd_gen)) # Output: (1, 'odd') print(next(even_odd_gen)) # Output: (2, 'even')
In this code snippet, the generator function even_odd_numbers()
generates both the number and its parity (even or odd) using multiple yield
statements. Each time the generator is iterated, it yields a tuple containing the number and its parity.
Code Snippet: Generator with Nested Generators
def outer_generator(): for i in range(3): yield i for j in range(2): yield (i, j) # Using the generator outer_gen = outer_generator() print(next(outer_gen)) # Output: 0 print(next(outer_gen)) # Output: (0, 0) print(next(outer_gen)) # Output: (0, 1)
This code snippet demonstrates a generator function outer_generator()
that contains a nested generator. The outer generator yields values from the outer loop, and the inner generator yields values from the inner loop. The generator object outer_gen
is then used to iterate over the generated values.
Code Snippet: Generator Chaining
def numbers(): yield from range(5) def squares(nums): for num in nums: yield num ** 2 # Using the generators num_gen = numbers() squares_gen = squares(num_gen) print(next(squares_gen)) # Output: 0 print(next(squares_gen)) # Output: 1 print(next(squares_gen)) # Output: 4
This code snippet demonstrates generator chaining, where the output of one generator is used as the input for another generator. The yield from
statement allows the squares()
generator to yield values from the numbers()
generator, effectively chaining the two generators together.
Related Article: How To Copy Files In Python
Code Snippet: Generator Expressions
squares_gen = (num ** 2 for num in range(5)) # Using the generator expression print(next(squares_gen)) # Output: 0 print(next(squares_gen)) # Output: 1 print(next(squares_gen)) # Output: 4
This code snippet demonstrates the use of generator expressions, which are concise ways to create generators. Generator expressions are similar to list comprehensions, but instead of creating a list, they create a generator that yields values on-the-fly.
Use Cases for Python Generators
Code Snippet: Generator for File Processing
def process_file(file_path): with open(file_path, "r") as file: for line in file: yield line.strip() # Using the generator file_gen = process_file("data.txt") for line in file_gen: print(line)
In this code snippet, the generator function process_file()
reads a file line by line and yields each line as a value. This allows you to process large files line by line without loading the entire file into memory.
Code Snippet: Generator for Data Streaming
def stream_data(data_source): for data in data_source: yield process_data(data) # Using the generator data_gen = stream_data(data_source) for processed_data in data_gen: print(processed_data)
This code snippet demonstrates a generator function stream_data()
that processes a stream of data. The function reads data from a data source (e.g., a network socket or a file) and yields the processed data. This allows you to process data in real-time as it becomes available.
Related Article: How to Append to a Dict in Python
Code Snippet: Generator for Infinite Sequences
def fibonacci(): a, b = 0, 1 while True: yield a a, b = b, a + b # Using the generator fib_gen = fibonacci() for _ in range(10): print(next(fib_gen))
This code snippet demonstrates a generator function fibonacci()
that generates an infinite sequence of Fibonacci numbers. The generator continues to yield Fibonacci numbers indefinitely, allowing you to generate as many numbers as needed without consuming excessive memory.
Best Practices for Using Python Generators
Code Snippet: Generator with Unpacking
def coordinates(): yield 10, 20, 30 # Using the generator with unpacking x, y, z = next(coordinates()) print(x, y, z) # Output: 10 20 30
In this code snippet, the generator function coordinates()
yields a tuple containing three values. The next()
function is used to retrieve the next value from the generator, and the tuple is unpacked into separate variables.
Code Snippet: Generator with Context Managers
from contextlib import contextmanager @contextmanager def open_file(file_path): file = open(file_path, "r") try: yield file finally: file.close() # Using the generator with a context manager with open_file("data.txt") as file: for line in file: print(line)
This code snippet demonstrates a generator function open_file()
that wraps a file object in a context manager. The yield
statement is used to define the point at which the code inside the with
block is executed. The file is automatically closed when the with
block is exited.
Related Article: How To Update A Package With Pip
Real World Examples of Python Generators
Code Snippet: Generator for Web Scraping
import requests def scrape_web_pages(urls): for url in urls: response = requests.get(url) yield response.text # Using the generator for web scraping url_list = ["https://example.com", "https://example.org"] page_gen = scrape_web_pages(url_list) for page in page_gen: print(page)
In this code snippet, the generator function scrape_web_pages()
takes a list of URLs and uses the requests library to fetch the web pages. The generator yields the HTML content of each web page, allowing you to scrape multiple web pages without loading them all into memory at once.
Code Snippet: Generator for Database Queries
import sqlite3 def query_database(query, params): conn = sqlite3.connect("database.db") cursor = conn.cursor() cursor.execute(query, params) while True: row = cursor.fetchone() if row is None: break yield row conn.close() # Using the generator for database queries select_query = "SELECT * FROM users WHERE age > ?" params = (18,) user_gen = query_database(select_query, params) for user in user_gen: print(user)
This code snippet demonstrates a generator function query_database()
that executes a SQL query on a SQLite database. The generator yields each row fetched from the database, allowing you to process large result sets without loading them all into memory.
Performance Considerations when Using Generators
Related Article: Working with Numpy Concatenate
Code Snippet: Generator with Lazy Evaluation
def fibonacci(): a, b = 0, 1 while True: yield a a, b = b, a + b # Using the generator lazily fib_gen = fibonacci() for _ in range(10): next(fib_gen)
In this code snippet, the Fibonacci generator is used in a way that demonstrates lazy evaluation. Only the first 10 Fibonacci numbers are generated and consumed, and the remaining numbers are never calculated. This lazy evaluation can improve performance when working with large or infinite sequences.
Code Snippet: Generator with Memory Efficiency
def read_large_file(file_path): with open(file_path, "r") as file: for line in file: yield line # Using the generator with memory efficiency file_gen = read_large_file("large_file.txt") for line in file_gen: process_line(line)
This code snippet demonstrates how generators can help improve memory efficiency when working with large files. Instead of reading the entire file into memory, the generator function read_large_file()
reads and yields one line at a time. This allows you to process large files without consuming excessive memory.
Code Snippet: Generator with Parallel Processing
from multiprocessing import Pool def process_item(item): # Process the item return processed_item def process_items(items): with Pool() as pool: for processed_item in pool.imap_unordered(process_item, items): yield processed_item # Using the generator with parallel processing items = get_items() item_gen = process_items(items) for processed_item in item_gen: print(processed_item)
This code snippet demonstrates how generators can be combined with parallel processing using the multiprocessing module. The process_items()
function uses a multiprocessing Pool to distribute the processing of items across multiple processes. The generator yields the processed items as they become available, allowing you to process items in parallel while consuming the results sequentially.
Error Handling in Python Generators
Related Article: How To Rename A File With Python
Code Snippet: Generator with Exception Handling
def divide_numbers(numbers): for num in numbers: try: yield 100 / num except ZeroDivisionError: yield "Error: Division by zero" # Using the generator with exception handling number_gen = divide_numbers([10, 5, 0, 20]) for result in number_gen: print(result)
In this code snippet, the generator function divide_numbers()
divides each number in the input list by 100. If a ZeroDivisionError occurs, the generator yields an error message instead of raising an exception. This allows you to handle errors gracefully and continue processing.
Code Snippet: Generator with Error Propagation
def process_data(data): try: yield process_step1(data) yield process_step2(data) yield process_step3(data) except ValueError as e: yield "Error: " + str(e) # Using the generator with error propagation data_gen = process_data(data) for result in data_gen: print(result)
This code snippet demonstrates a generator function process_data()
that performs multiple processing steps on the input data. If a ValueError occurs during any of the processing steps, the generator yields an error message instead of raising an exception. This allows you to propagate errors through the generator and handle them at a higher level.
Pros and Cons of Using Python Generators
Python generators offer several advantages and disadvantages. Here are some pros and cons to consider when using generators:
Pros:
- Memory efficiency: Generators allow you to process large or infinite sequences without consuming excessive memory.
- Lazy evaluation: Generators only generate values as needed, allowing for efficient processing of sequences.
- Simplified code: Generators can make code more concise and readable, especially when working with complex sequences.
- Seamless integration: Generators can be used in a variety of contexts, such as for loop iteration, list comprehension, and function composition.
Cons:
- Limited random access: Unlike lists or arrays, generators do not support random access to elements. You can only iterate over the values in a sequential manner.
- One-time use: Generators can only be iterated once. Once all the values have been generated and consumed, the generator is exhausted.
- Performance overhead: The use of generators introduces some performance overhead compared to direct iteration over data structures like lists.
Despite these limitations, Python generators are a valuable tool for efficiently working with sequences and generating values on-the-fly. By understanding their capabilities and limitations, you can leverage generators to write more efficient and readable code.