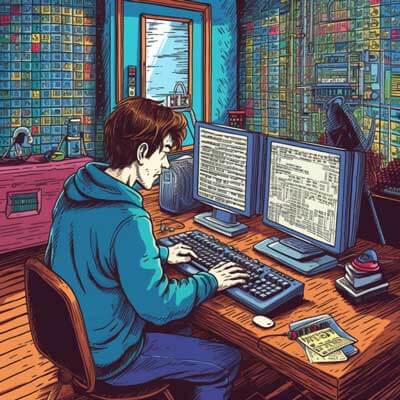
Table of Contents
How can I configure webpack to use the Awesome TypeScript Loader?
To configure webpack to use the Awesome TypeScript Loader, you need to install the necessary dependencies and update your webpack configuration file.
Here are the steps to configure webpack to use the Awesome TypeScript Loader:
1. Install the required dependencies:
npm install typescript awesome-typescript-loader --save-dev
2. Update your webpack configuration file (usually named webpack.config.js
) to include the loader configuration. Add a new rule for TypeScript files that uses the awesome-typescript-loader
. Here's an example configuration:
module.exports = { // ... other webpack configuration options module: { rules: [ // ... other rules { test: /\.tsx?$/, exclude: /node_modules/, use: { loader: 'awesome-typescript-loader', }, }, ], }, resolve: { extensions: ['.js', '.jsx', '.ts', '.tsx'], }, };
This configuration tells webpack to use the awesome-typescript-loader
for all files with the .ts
or .tsx
extension. The loader will transpile the TypeScript code into JavaScript code, which can then be processed by other loaders or bundled into the final output.
3. Update your entry file(s) to use the appropriate file extension. For example, if your entry file is main.ts
, update it to main.tsx
if it contains JSX syntax.
With these configuration changes, webpack will now use the Awesome TypeScript Loader to transpile your TypeScript code during the build process.
Related Article: Tutorial: Using React-Toastify with TypeScript
Code Snippet: Installing TypeScript
To install TypeScript, you can use npm, the package manager for Node.js. Open your command line interface and run the following command:
npm install typescript --save-dev
This command installs TypeScript as a dev dependency in your project. The --save-dev
flag ensures that TypeScript is added to the devDependencies
section of your package.json
file.
Code Snippet: Configuring webpack
To configure webpack to use the Awesome TypeScript Loader, update your webpack configuration file (usually named webpack.config.js
). Add a new rule for TypeScript files that uses the awesome-typescript-loader
. Here's an example configuration:
module.exports = { // ... other webpack configuration options module: { rules: [ // ... other rules { test: /\.tsx?$/, exclude: /node_modules/, use: { loader: 'awesome-typescript-loader', }, }, ], }, resolve: { extensions: ['.js', '.jsx', '.ts', '.tsx'], }, };
This configuration tells webpack to use the awesome-typescript-loader
for all files with the .ts
or .tsx
extension. The loader will transpile the TypeScript code into JavaScript code, which can then be processed by other loaders or bundled into the final output.
Code Snippet: Creating a TypeScript file
To create a TypeScript file, simply create a new file with a .ts
or .tsx
extension and start writing TypeScript code. Here's an example of a simple TypeScript file that defines a function:
// greeting.ts function sayHello(name: string) { console.log(`Hello, ${name}!`); } sayHello("TypeScript");
This TypeScript file defines a function sayHello
that takes a name
parameter of type string
and logs a greeting to the console. The function is then called with the argument "TypeScript"
.
Related Article: Fixing 'TypeScript Does Not Exist on Type Never' Errors
Code Snippet: Using the Awesome TypeScript Loader
Once you have configured webpack to use the Awesome TypeScript Loader, you can import and use TypeScript files in your JavaScript code. Here's an example of how to import a TypeScript module and use a function defined in it:
// index.js import { sayHello } from './greeting'; sayHello("TypeScript");
In this example, the JavaScript file index.js
imports the sayHello
function from the TypeScript module greeting.ts
. The function is then called with the argument "TypeScript"
. The Awesome TypeScript Loader will transpile the TypeScript code into JavaScript code, which can be executed by the browser.
Code Snippet: Building and running the project
To build and run the project with webpack, you can use the webpack
command-line tool. By default, webpack will look for a configuration file named webpack.config.js
in the current directory. Here are the steps to build and run the project:
1. Run the following command in your project directory to build the project:
npx webpack
This command will execute webpack with the default configuration file (webpack.config.js
) and bundle your code according to the specified rules.
2. After the build process completes, you can run the project by opening the generated HTML file in a web browser. The bundled JavaScript code will be loaded and executed by the browser, and your application should be up and running.
Common Errors: TypeScript configuration issues
When working with TypeScript and webpack, you may encounter some common configuration issues. Here are a few examples:
1. Error: Module not found: Error: Can't resolve 'module' in '...'
This error occurs when webpack cannot find a module that is being imported in your TypeScript code. Make sure that the module is installed and that the import path is correct. You may need to update your TypeScript configuration or webpack resolve settings to include the correct module paths.
2. Error: Property '...' does not exist on type '...'
TypeScript is a statically typed language, and it performs strict type checking. This error occurs when you try to access a property or call a method that does not exist on a given type. Make sure that the types in your code are correctly defined and that you are using them appropriately.
3. Error: Cannot find module '.../...'
This error occurs when TypeScript or webpack cannot find a module that is being imported in your code. Make sure that the module is installed and that the import path is correct. You may need to update your TypeScript configuration or webpack resolve settings to include the correct module paths.
Common Errors: Loader configuration issues
When configuring loaders in webpack, you may encounter some common issues. Here are a few examples:
1. Error: Module build failed: Error: You may need an appropriate loader to handle this file type.
This error occurs when webpack cannot find a loader for a specific file type. Make sure that the necessary loaders are installed and correctly configured in your webpack configuration file. Check the file extension and the corresponding loader configuration in your rules.
2. Error: Module build failed: SyntaxError: Unexpected token
This error occurs when webpack encounters a syntax error in one of the files being processed by a loader. Check the file that is causing the error and make sure that it is written correctly. You may need to update your loader configuration or use a different loader to handle the specific file type.
3. Error: Module build failed: Error: Cannot find module '.../...'
This error occurs when webpack cannot find a necessary module or dependency that is required by one of the loaders. Make sure that all the required dependencies are installed and that the import paths in your loader configuration are correct.
Related Article: How to Iterate Through a Dictionary in TypeScript
Common Errors: Transpilation errors
When transpiling TypeScript code to JavaScript, you may encounter some common errors. Here are a few examples:
1. Error: Cannot redeclare block-scoped variable '...'
This error occurs when you declare a variable with the same name multiple times within the same block scope. Make sure that your variable names are unique and that you are not redeclaring them.
2. Error: Property '...' does not exist on type '...'
This error occurs when you try to access a property or call a method that does not exist on a given type. Make sure that your types are correctly defined and that you are using them appropriately. Check the TypeScript documentation or the typings for the libraries you are using.
3. Error: Type '...' is not assignable to type '...'
This error occurs when you try to assign a value of one type to a variable or parameter of a different incompatible type. Make sure that you are assigning values of the correct type and that your type annotations are correct.
What is a loader in the context of webpack?
In the context of webpack, a loader is a module that transforms or processes a specific type of file. Loaders are used by webpack to handle different file types and apply transformations to them before bundling them into the final output. For example, the TypeScript loader is used to transpile TypeScript code into JavaScript code, while the CSS loader is used to process CSS files and extract styles from them.
Loaders are specified in the webpack configuration file using rules. Each rule defines a set of conditions that must be met for a file to be processed by a specific loader. Loaders can be chained together, allowing for complex transformations and processing of files. Webpack provides a wide range of built-in loaders, and developers can also create their custom loaders to handle specific file types or apply custom transformations.
What is the difference between TypeScript and JavaScript?
TypeScript and JavaScript are both programming languages that are used for web development, but they have some key differences.
JavaScript is a dynamic, interpreted language that is primarily used for client-side scripting in web browsers. It is a widely supported language and runs on virtually all modern web browsers and platforms. JavaScript code is executed directly by the browser without any additional processing.
TypeScript, on the other hand, is a statically typed superset of JavaScript. It adds optional static typing, classes, interfaces, and other features to JavaScript, making it more suitable for large-scale application development. TypeScript code needs to be transpiled into JavaScript before it can be executed by a browser or a JavaScript runtime.
One of the main advantages of TypeScript is its ability to catch common errors and provide better tooling support, thanks to its static typing. It allows developers to catch type-related bugs during development and provides better code organization and maintainability. TypeScript also supports the latest ECMAScript features and provides advanced features like decorators and generics.
What is a module bundler?
A module bundler is a tool that combines multiple modules or files into a single file or a set of files that can be loaded by a web browser. It helps manage dependencies between modules and optimizes the loading and execution of code.
In modern web development, applications are often built using a modular approach, where functionality is encapsulated into separate modules that can be developed and tested independently. However, web browsers do not natively support modules, and loading multiple individual script files can be inefficient and slow.
A module bundler, such as webpack, addresses these challenges by analyzing the dependencies between modules and bundling them together into a single file or a set of files. This allows for better code organization, improved performance, and easier deployment. Module bundlers can also apply transformations and optimizations to the bundled code, such as minification, dead code elimination, and tree shaking, to further improve the size and performance of the application.
Related Article: How to Verify if a Value is in Enum in TypeScript
What is the role of a build process in frontend development?
The build process plays a crucial role in frontend development by transforming and optimizing the source code of a web application into a form that can be executed by a web browser. It encompasses various tasks, such as transpiling code, bundling modules, optimizing assets, and generating deployment-ready artifacts.
The build process allows developers to write code in languages and formats that are not natively supported by web browsers, such as TypeScript, ES6, and SCSS. It enables the use of modern language features and tools that improve productivity, code organization, and maintainability.
In addition to transpiling and bundling code, the build process can also perform other tasks, such as optimizing assets like images and fonts, generating sourcemaps for easier debugging, and injecting environment-specific configuration values. It can also run tests, lint code for common errors and best practices, and generate documentation.
The output of the build process is typically a set of static files that can be deployed to a web server or a content delivery network (CDN). These files include the bundled JavaScript and CSS code, along with any other assets needed by the application, such as images, fonts, and HTML templates.
Build processes can be configured using build tools, such as webpack, Gulp, or Grunt, which provide a flexible and extensible way to define and automate the various tasks involved in the build process.
What does a transpiler do?
A transpiler, also known as a source-to-source compiler, is a tool that translates code written in one programming language into code written in another programming language. In the context of frontend development, transpilers are commonly used to convert code written in languages like TypeScript or ES6 into code that can be executed by web browsers, which typically only understand plain JavaScript.
Transpilers work by parsing the source code written in the input language, analyzing its structure and meaning, and then generating equivalent code in the output language. This process often involves transforming and rewriting the code to ensure that it conforms to the syntax and semantics of the output language.
For example, a TypeScript transpiler takes TypeScript code as input and generates equivalent JavaScript code as output. The transpiler performs type checking, static analysis, and other optimizations to ensure that the resulting JavaScript code is correct and efficient.
Transpilers are an essential part of modern frontend development workflows, as they allow developers to write code in higher-level languages that provide advanced features and better tooling support. They enable the use of modern language features and syntax that are not natively supported by web browsers, while still ensuring compatibility and performance.
What are type annotations in TypeScript?
Type annotations in TypeScript are a way to specify the type of a variable, function parameter, or function return value. They provide static type checking, which helps catch type-related errors during development and improves code quality and maintainability.
Type annotations in TypeScript use a syntax similar to JavaScript's type system, but with some additional features and enhancements. For example, basic types like number
, string
, and boolean
can be used as type annotations, as well as more advanced types like arrays, objects, and custom types.
Here are some examples of type annotations in TypeScript:
let count: number = 10; let message: string = "Hello, TypeScript!"; let isActive: boolean = true; function add(a: number, b: number): number { return a + b; } interface Person { name: string; age: number; } let person: Person = { name: "John Doe", age: 25, };
Type annotations allow the TypeScript compiler to perform static type checking, which means it can detect type-related errors at compile-time. This helps catch common programming mistakes and provides better tooling support, such as autocompletion and type inference, which can improve developer productivity.
External Sources