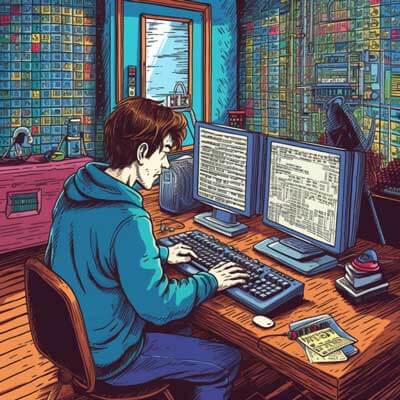
Table of Contents
How to Access State in React
In React, state is used to manage the data that is specific to a component. It allows components to store and manage data that can change over time. To access the state in React, you can use the this.state
syntax within the component class. Here is an example:
class MyComponent extends React.Component { constructor(props) { super(props); this.state = { count: 0 }; } render() { return ( <div> <p>Count: {this.state.count}</p> </div> ); } }
In the above example, the count
property is accessed using this.state.count
. This allows you to display the value of count
in the component's render method.
Related Article: Implementing Server Rendering with Ruby on Rails & ReactJS
What is the Purpose of the setState Method
The setState
method is used to update the state of a component in React. It allows you to update the value of a state property and trigger a re-render of the component. The setState
method is asynchronous, which means that React may batch multiple setState
calls together for performance reasons.
Here is an example of how to use the setState
method:
class MyComponent extends React.Component { constructor(props) { super(props); this.state = { count: 0 }; } handleClick() { this.setState({ count: this.state.count + 1 }); } render() { return ( <div> <p>Count: {this.state.count}</p> <button onClick={() => this.handleClick()}>Increment</button> </div> ); } }
In the above example, the handleClick
method updates the count
property of the state using the setState
method. When the button is clicked, the handleClick
method is called, which increments the count
property by 1. The updated state triggers a re-render of the component, causing the updated count to be displayed.
How Can I Update a State Property in React
To update a state property in React, you can use the setState
method. When calling setState
, you can pass in an object that represents the updated state. React will merge this object with the current state and trigger a re-render of the component.
Here is an example of updating a state property in React:
class MyComponent extends React.Component { constructor(props) { super(props); this.state = { name: 'John', age: 25 }; } handleInputChange(event) { this.setState({ name: event.target.value }); } render() { return ( <div> <input type="text" value={this.state.name} onChange={(event) => this.handleInputChange(event)} /> <p>Name: {this.state.name}</p> <p>Age: {this.state.age}</p> </div> ); } }
In the above example, the handleInputChange
method is called whenever the input field's value changes. It updates the name
property of the state with the new value entered in the input field. The updated state triggers a re-render of the component, causing the updated name to be displayed.
Best Practices for State Management in React
When it comes to state management in React, there are some best practices that can help you write cleaner and more maintainable code:
1. Keep state as minimal as possible: Only store the data that is necessary for the component's functionality in the state. If a piece of data is not needed for rendering or component logic, it can be stored as a regular variable outside of the state.
2. Use functional setState when updating state based on previous state: When updating state based on its previous value, it is recommended to use the functional form of setState
to ensure that the update is based on the latest state. Here is an example:
this.setState((prevState) => ({ count: prevState.count + 1 }));
3. Avoid mutating state directly: React expects state to be immutable, so you should not directly mutate the state object. Instead, create a new object with the updated values and use setState
to update the state. This ensures that React can properly track changes and trigger re-renders.
4. Lift state up when needed: If multiple components need access to the same piece of state or need to update the same state property, it is recommended to lift the state up to a common ancestor component. This allows the components to share the state and keeps it in sync across the application.
5. Use controlled components for user input: In React, controlled components are components where the value of the input element is controlled by the state. This allows you to have full control over the input value and perform validations or transformations before updating the state.
Related Article: How to Install Webpack in ReactJS: A Step by Step Process
How to Pass Props to a Child Component in React
In React, props are used to pass data from a parent component to its child components. This allows components to communicate and share data with each other. To pass props to a child component, you can simply include them as attributes when rendering the child component.
Here is an example of passing props to a child component in React:
class ParentComponent extends React.Component { render() { const name = 'John'; const age = 25; return ( <div> <ChildComponent name={name} age={age} /> </div> ); } } class ChildComponent extends React.Component { render() { return ( <div> <p>Name: {this.props.name}</p> <p>Age: {this.props.age}</p> </div> ); } }
In the above example, the ParentComponent
renders the ChildComponent
and passes the name
and age
props to it. The ChildComponent
then receives these props as properties of its props
object and can access them using this.props.name
and this.props.age
.
You can pass any type of data as props, including strings, numbers, booleans, arrays, objects, and even functions.
What Happens When a Component's State is Updated in React
When a component's state is updated in React, several things happen behind the scenes:
1. React merges the updated state: When you call setState
to update the state, React merges the updated state with the current state. It only updates the properties that are explicitly changed in the new state object, leaving the other properties unchanged.
2. React triggers a re-render: After merging the updated state, React triggers a re-render of the component. This means that the component's render
method is called again and the updated state is used to generate the new UI.
3. React updates the virtual DOM: During the re-render, React updates the virtual DOM, which is an in-memory representation of the actual DOM. React compares the new virtual DOM with the previous virtual DOM and determines the minimal set of changes needed to update the actual DOM.
4. React applies the DOM updates: Finally, React applies the updates to the actual DOM, making the necessary changes to reflect the updated state. This includes adding, removing, or updating DOM elements based on the changes determined in the virtual DOM.
It's important to note that React performs these operations efficiently by using a diffing algorithm to minimize the number of DOM updates. This helps to optimize the performance of React applications by reducing unnecessary DOM manipulations.
How Does React Handle Re-rendering After a State Update
When a component's state is updated in React, React handles the re-rendering process efficiently by performing a reconciliation process. Here is how React handles re-rendering after a state update:
1. State update triggers re-render: When you call setState
to update the state, React marks the component as dirty and schedules a re-render of the component.
2. Virtual DOM comparison: During the re-render, React creates a new virtual DOM representation of the component's UI based on the updated state and the component's render method. React then compares this new virtual DOM with the previous virtual DOM to determine the differences.
3. Diffing algorithm: React uses a diffing algorithm to efficiently determine the minimal set of changes needed to update the actual DOM. This algorithm compares the new virtual DOM with the previous virtual DOM and identifies the added, removed, and updated elements.
4. Batched updates: To optimize performance, React batches multiple state updates together. This means that if you call setState
multiple times within the same event loop, React will batch these updates together and perform a single re-render. This helps to avoid unnecessary re-renders and improves the performance of React applications.
5. Efficient DOM updates: After determining the differences between the new and previous virtual DOM, React applies the necessary DOM updates to reflect the updated state. React uses an efficient algorithm to update only the affected elements in the actual DOM, minimizing the impact on performance.
The Role of the Render Method in React
In React, the render
method plays a crucial role in defining the UI of a component. It is a required method that must be implemented in every class-based component. The render
method returns a React element, which is a lightweight representation of the component's UI.
Here is an example of a simple component with a render
method:
class MyComponent extends React.Component { render() { return ( <div> <p>Hello, World!</p> </div> ); } }
In the above example, the render
method returns a JSX expression that represents the component's UI. This JSX is then transformed into JavaScript code by a process called transpilation, allowing React to create and update the actual DOM elements.
The render
method is called whenever the component needs to be re-rendered, which can happen due to state changes, prop updates, or parent component re-renders. React determines when to call the render
method based on the component's state and props.
It's important to note that the render
method should be pure, meaning that it should not modify the component's state or have any side effects. It should only return a new React element based on the current state and props of the component. This ensures that the render method is predictable and can be safely called multiple times.
Related Article: How to Fetch and Post Data to a Server Using ReactJS
Updating Multiple State Properties at Once in React
In React, you can update multiple state properties at once by passing an object to the setState
method. This object should contain all the state properties that you want to update. React will merge this object with the current state and trigger a re-render of the component.
Here is an example of updating multiple state properties at once in React:
class MyComponent extends React.Component { constructor(props) { super(props); this.state = { count: 0, name: 'John' }; } handleClick() { this.setState({ count: this.state.count + 1, name: 'Jane' }); } render() { return ( <div> <p>Count: {this.state.count}</p> <p>Name: {this.state.name}</p> <button onClick={() => this.handleClick()}>Update</button> </div> ); } }
In the above example, the handleClick
method updates both the count
and name
properties of the state using the setState
method. When the button is clicked, the handleClick
method is called, which increments the count
property by 1 and updates the name
property to 'Jane'. The updated state triggers a re-render of the component, causing the updated count and name to be displayed.
How to Update State Based on Previous State in React
In React, it is a common requirement to update the state based on its previous value. To achieve this, you can use the functional form of the setState
method. This allows you to access the previous state and perform calculations or transformations before updating the state.
Here is an example of updating the state based on its previous value in React:
class MyComponent extends React.Component { constructor(props) { super(props); this.state = { count: 0 }; } handleIncrement() { this.setState((prevState) => ({ count: prevState.count + 1 })); } handleDecrement() { this.setState((prevState) => ({ count: prevState.count - 1 })); } render() { return ( <div> <p>Count: {this.state.count}</p> <button onClick={() => this.handleIncrement()}>Increment</button> <button onClick={() => this.handleDecrement()}>Decrement</button> </div> ); } }
In the above example, the handleIncrement
and handleDecrement
methods update the count
property of the state based on its previous value. By using the functional form of setState
, we can access the previous state (prevState
) and increment or decrement the count
property accordingly. This ensures that the state is updated correctly even if multiple updates are triggered in quick succession.