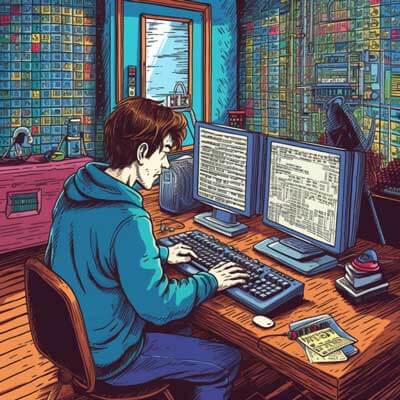
Table of Contents
Overview of Nested Lists
A list is a data structure that allows you to store multiple values in a single variable. A nested list, also known as a list of lists, is a list that contains other lists as its elements. This allows you to create multidimensional arrays or matrices in Python.
A nested list can be visualized as a grid, where each element in the outer list represents a row, and each element in the inner lists represents a column. This can be useful when working with tabular data or when you need to represent a grid-like structure in your code.
Related Article: How to Use Collections with Python
Working with Multidimensional Lists
Python provides a simple and intuitive way to create and work with multidimensional lists. You can create a nested list by enclosing multiple lists within square brackets, separated by commas. For example:
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
In this example, matrix
is a nested list with three inner lists, each containing three elements. You can access individual elements in a nested list by using multiple indices. For example, to access the element at the second row and third column of the matrix
, you can use the following syntax:
element = matrix[1][2]
This will return the value 6
, as indexing in Python starts from 0.
2D Arrays
A two-dimensional (2D) array is a specific type of nested list where all the inner lists have the same length. This can be useful when you need to represent a grid or a table of values. In Python, you can create a 2D array by initializing a nested list with a consistent number of elements in each inner list.
Here's an example of a 2D array representing a 3x3 grid:
grid = [[0, 0, 0], [0, 0, 0], [0, 0, 0]]
You can access and modify individual elements in a 2D array using the same indexing syntax as for nested lists. For example, to change the value at the second row and third column of the grid
to 1
, you can use the following code:
grid[1][2] = 1
Accessing Elements in a List of Lists
To access elements in a list of lists, you need to use nested indexing. The first index refers to the outer list, and the second index refers to the inner list.
For example, consider the following list of lists:
data = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
To access the element at the second row and third column, you can use the following code:
element = data[1][2]
This will return the value 6
.
You can also use negative indexing to access elements from the end of the list. For example, to access the last element in the last inner list, you can use the following code:
last_element = data[-1][-1]
This will return the value 9
.
Related Article: Optimizing FastAPI Applications: Modular Design, Logging, and Testing
Advantages of Using a List of Lists
Using a list of lists in Python has several advantages. Firstly, it allows you to create and manipulate multidimensional data structures, such as grids or matrices, in a straightforward manner. This can be especially useful when working with scientific or numerical data.
Secondly, a list of lists provides a flexible way to represent data that has varying lengths. Each inner list can have a different number of elements, allowing you to handle situations where the data is not uniform. This is in contrast to other data structures, such as NumPy arrays, which require a fixed shape.
Lastly, a list of lists can be easily modified and updated. You can add or remove elements from the inner lists, change the length of the inner lists, or even add or remove entire inner lists. This flexibility makes lists of lists a versatile choice for many programming tasks.
Managing Different Lengths in a List of Lists
In a list of lists, it is possible to have inner lists of different lengths. This can be useful when you need to represent data that is not uniform, such as a table with varying numbers of columns.
To manage different lengths in a list of lists, you can use Python's built-in list methods. For example, to add a new element to an inner list, you can use the append()
method. To remove an element from an inner list, you can use the pop()
method.
Here's an example that demonstrates how to manage different lengths in a list of lists:
data = [[1, 2, 3], [4, 5], [6, 7, 8, 9]] # Add an element to the second inner list data[1].append(6) # Remove the last element from the third inner list data[2].pop() print(data)
Output:
[[1, 2, 3], [4, 5, 6], [6, 7, 8]]
In this example, the second inner list has been updated to include the element 6
, and the last element in the third inner list has been removed.
Additional Resources
- Advantages of using a list of lists in Python